Thanks to the Canvas element of HTML5, complex drawings and drawing functions are possible in HTML pages. This opens up new possibilities for online forms, such as the implementation of a signature function.
In our example we want to create a form that allows the user to create a signature (or any other drawing). Optionally with mouse or on mobile devices with touch functions.
The form is then processed by a PHP script. Our example will look like this:
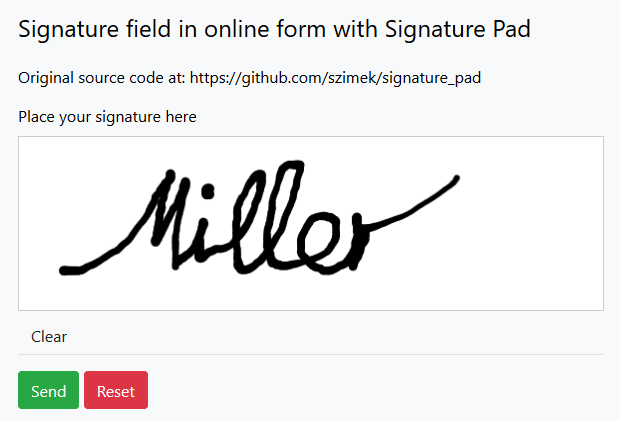
As you can already see in the screenshot, we do not want to completely reinvent the wheel, but use an open source component for the basic functions. The component uses different stroke widths when drawing, so that the impression of a real pen or signature is created.
Let’s do it. The whole example is available for download at the end. The first step is to create an HTML file. There we add the Java-Script library for the signature to the closing body tag:
<script src="signature_pad.js"></script>
We then define the elements for the signature field in our form:
<div id="signature-pad" class="m-signature-pad"> <div class="m-signature-pad--body"> <canvas></canvas> <input type="hidden" name="signature" id="signature" value=""> </div> </div>
We see here an empty canvas, which will later realize our signature field. There is also a hidden form field, which we use to transfer the data to our PHP script.
For the definition of our design we use some CSS code:
<style type="text/css"> .m-signature-pad--body canvas { position: relative; left: 0; top: 0; width: 100%; height: 250px; border: 1px solid #CCCCCC; } </style>
Here we can define width, height and frame color. Now we have to “wire” the canvas with the corresponding functions:
var wrapper = document.getElementById("signature-pad"), canvas = wrapper.querySelector("canvas"), signaturePad; var signaturePad = new SignaturePad(canvas); signaturePad.minWidth = 1; //minimale Breite des Stiftes signaturePad.maxWidth = 5; //maximale Breite des Stiftes signaturePad.penColor = "#000000"; //Stiftfarbe signaturePad.backgroundColor = "#FFFFFF"; //Hintergrundfarbe
This connects our canvas with the Java-Script of the component and now allows drawing into the canvas. In addition, we can set various properties such as colors, pen thickness and background color.
Now the drawing works, but does not react optimally to changes in size of the browser. Therefore we still have to implement the handling of resizing the browser:
function resizeCanvas() { var oldContent = signaturePad.toData(); var ratio = Math.max(window.devicePixelRatio || 1, 1); canvas.width = canvas.offsetWidth * ratio; canvas.height = canvas.offsetHeight * ratio; canvas.getContext("2d").scale(ratio, ratio); signaturePad.clear(); signaturePad.fromData(oldContent); }
We assign this function to the event of the browser window and also call the method once with the first call:
window.onresize = resizeCanvas; resizeCanvas();
What’s the point of all this? When the browser is resized, the content of the canvas changes, may even be deleted, compressed etc. Therefore we save the original content, determine the new size and place the old content into the enlarged or reduced canvas.
What is still missing now is the transmission of the signature to a processing PHP script. The canvas element is not transferred when the form is submitted. Therefore we save the content in Data-URL format into the hidden field:
function submitForm() { //Unterschrift in verstecktes Feld übernehmen document.getElementById('signature').value = signaturePad.toDataURL(); }
The component has a handy feature that allows us to get the image content back directly as Data-URL. We now have to call this function before sending the form, this can be done by the corresponding event of the form:
<form class="w3-container" action="process.php" method="POST" name="DAFORM" onSubmit="submitForm();" enctype="multipart/form-data" target="_self">
Done. When sending, we save the signature or the image in ASCII format in the hidden field. There we will find the following content in the PHP script:
data:image/png;base64,iVBORw0KGgoAAAAN…
The advantage of the Data-URL format? Well we can use the content directly to display the image in an image tag:
<img src="data:image/png;base64,iVBORw0KGgoAAAAN...">
We now make use of this in the PHP script. Here we simply read out the POST variable of the form and output the image:
$image = ""; if (isset($_POST["signature"])) { $image = $_POST["signature"]; echo ""; } else { echo "
Kein Bild übertragen
“; }
The result:
And we have already created a form with signature or drawing function. In the example you will find the possibility to delete the field with a button, as well as to download the image directly in the browser as an image.
Download des Beispiels
The complete example is available here. Download the original component on Github.
Integration in DA-FormMaker
Another tip for those who find the whole thing too much manual work: The entire functionality of the drawing or signature field is already integrated in our software DA-FormMaker and DA-OrderForm. Any number of signature fields can be added to the forms. The configuration of colors, design, size are done directly in the software. Checks for empty fields are also possible:
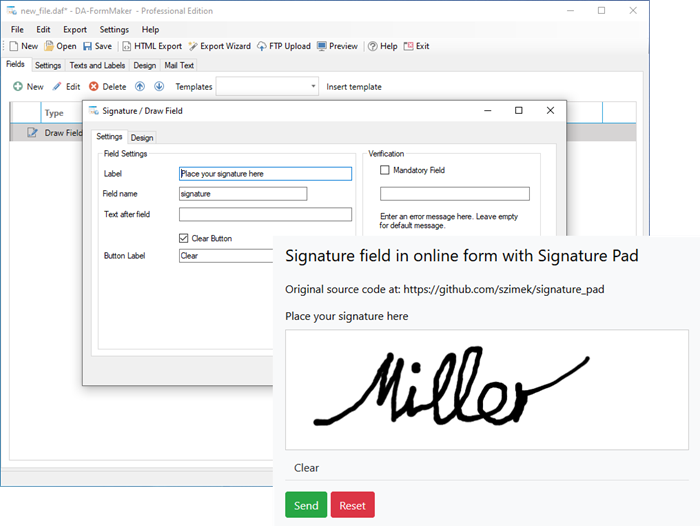
The code is generated automatically and forms with a signature field can be used immediately. When the form is submitted, the signature is displayed inline in the HTML e-mail. In addition, the drawing is inserted as a PNG file in the attachment.
Sorry I can get this to work, even the demo.
Whats the problem? Maybe you can upload an example and share it.
Hi,
I got everything working.
But I was wondering if you could tell me how to change the PHP script to send the image into email.
Great tutorial by the way.
Hi,
thanks for the feedback. If you want an example how you can add the signature to an email, you can have a look to the formmail script of our software DA-FormMaker: https://secure.da-software.de/DA-FormMaker/index.html
With our software you can create a signature, which will be added to the email. If you are interested in the details search for the “htmlgenerator.php” in the formmail script. A signature / image is transmitted as base64 to the script and added to the html email as CID (signature.php) image:
$sig = new SignatureItem($str);
$currentSignatureHtml = $sig->getCidImage();
Another option is so use a base64 image:
$currentSignatureHtml = $sig->getHtmlImage();
Best to have a look to the signature.php, to see how this works.
Andy
I’ve copied the code to my website, how do I then access people’s signatures? (I’m a beginner)
Cheers!
Hi, well that depends, usually you would integrate the field into a form and when the user hits the send button you have to process the data, for example with a PHP script. Depending on hwat you want to achieve, you can have a look to our product DA-FormMaker, which does this for you: https://da-software.net/en/software/da-formmaker/
Is there any way to make this field a required field?
Thanks!
Hi Valentin,
hi there is, you can have a look at our software DA-FormMaker, the software allows to set the signature field as mandatory, the code looks like this:
if (signaturePad0.isEmpty()) {
alert(“Error message”);
return false;
}
Andy